Dependency Injection (DI) in TypeScript
dependency-injection
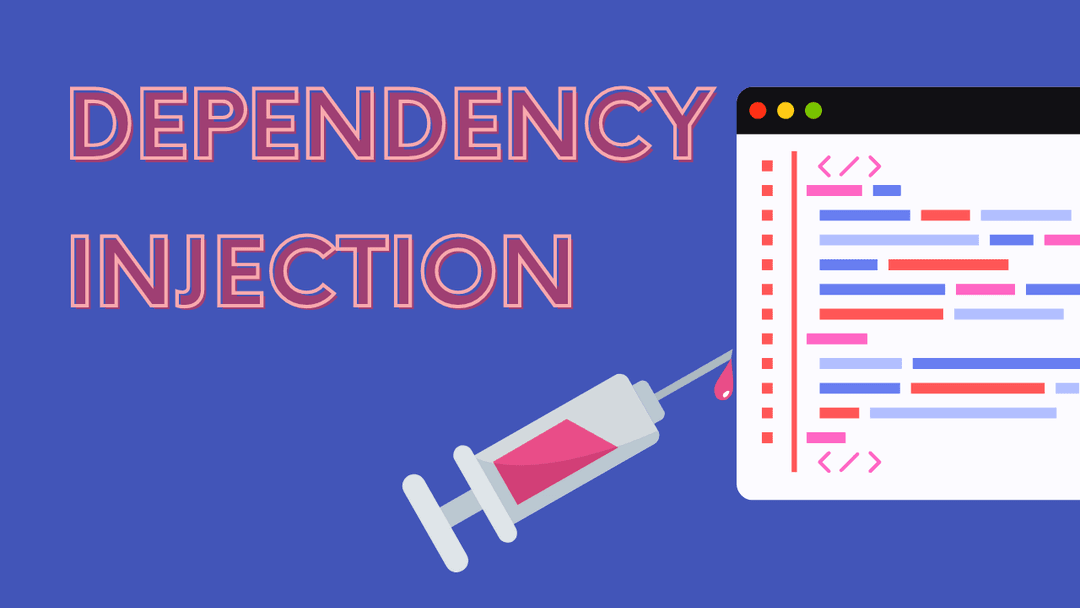
1. What is Dependency Injection (DI)?
Dependency Injection (DI) is a design pattern used in software development to provide objects or classes with the dependencies they need to function, rather than letting them create those dependencies themselves. This ensures that a class or object is not tightly bound to specific implementations, allowing for greater flexibility and modularity.
Think of it this way: DI is like giving a chef the ingredients they need to cook, rather than expecting them to grow the vegetables, raise livestock, and gather spices themselves.
2. Why Use Dependency Injection?
The main goal of DI is to avoid tight coupling between different parts of your application. When a class directly creates its dependencies, it becomes difficult to reuse, test, or change that class. Dependency Injection solves this by allowing classes to receive their dependencies from external sources, resulting in:
- Flexibility: Classes can work with different implementations of their dependencies without requiring code changes.
- Reusability: Dependencies can be shared across multiple classes, reducing duplication.
- Maintainability: Changing a dependency doesn’t require altering the classes that use it.
- Testability: Dependencies can be replaced with mocks or stubs for easier testing.
3. Dependency Injection and Inversion of Control (IoC)
DI is a practical application of Inversion of Control (IoC), a principle where the control of object creation and dependency management is shifted from the class itself to an external entity. Instead of a class being responsible for managing its own dependencies, it relies on an external source to provide them.
Imagine a delivery service where the driver doesn’t need to decide what package to deliver or where to deliver it. The logistics team manages those details, leaving the driver to focus solely on driving.
4. Types of Dependency Injection
Dependency Injection can be implemented in various ways, each with its own use case:
Constructor Injection
Dependencies are provided through a class’s constructor at the time of instantiation. This is ideal for dependencies that are essential for the class to function.
Setter Injection
Dependencies are assigned through setter methods after the class is created. This is useful for optional or replaceable dependencies.
Interface Injection
Dependencies are provided via an interface that the dependent class implements. This allows for more dynamic and flexible dependency management.
Each type has its own strengths, and the choice depends on the specific requirements of your application.
5. Advantages of Dependency Injection
Here are some key benefits of using Dependency Injection in your applications:
- Loose Coupling: Classes are independent of specific implementations, making it easier to swap or modify dependencies.
- Improved Testability: By injecting dependencies, you can easily replace them with mock versions for testing.
- Better Maintainability: Changes to dependencies don’t require modifying the classes that use them, reducing the risk of introducing bugs.
- Reusability: Classes can work with a wide range of dependencies, increasing their versatility.
- Enhanced Readability: DI makes it clear what a class depends on, improving code clarity and documentation.
6. Real-World Analogy
Let’s break down DI with a real-world example:
Imagine a car that requires an engine to operate. If the car itself is responsible for creating its engine, it becomes tightly coupled to that specific engine type. Now, if you want the car to use a different engine—say, an electric engine instead of a petrol engine—you’d need to modify the car's code.
With Dependency Injection, the car doesn’t create its own engine. Instead, it receives the engine from an external source. This means you can easily swap the petrol engine for an electric one without changing the car’s internal logic.
7. Practical Benefits in Software Development
In software development, DI offers solutions to several common challenges:
- Swapping Dependencies: Imagine a web application that connects to a database. With DI, you can switch between a development database and a production database without altering the application’s core logic.
- Testing: DI allows you to inject mock services, making it easy to test different parts of your application in isolation.
- Scalability: As your application grows, managing dependencies manually can become cumbersome. DI simplifies this by centralizing dependency management, making the application easier to scale and maintain.
8. Key Takeaways
- Dependency Injection is an essential design pattern that ensures loose coupling between classes and their dependencies.
- It improves flexibility, testability, and maintainability, making it a crucial practice for professional-grade applications.
- In TypeScript, DI can be implemented in multiple ways, such as constructor injection, setter injection, and interface injection.
- Real-world examples, like swapping engines in a car or changing database connections in a web app, demonstrate the power and versatility of DI.
By understanding and applying Dependency Injection, developers can build more robust, maintainable, and scalable applications. Whether you’re a beginner or a seasoned developer, mastering DI is a step toward writing cleaner and more professional code.
Leave a Comment
No comments yet. Be the first to comment!