Design Pattern In Software Engineering
design-patterns
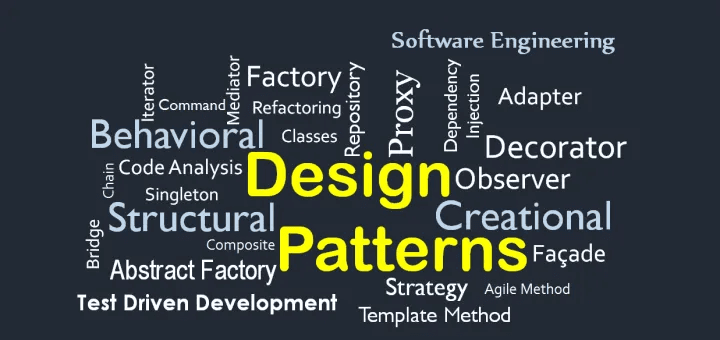
1. Factory Pattern: Simplifying Object Creation
Use Case: A food delivery application needs to create different types of user accounts (e.g., customer, restaurant owner, delivery driver). Each user type has different properties and behaviors.
In functional programming, a factory pattern can be represented as a function that returns the appropriate user object based on input parameters. This avoids complex conditional logic spread throughout the application and centralizes the creation logic.
2. Singleton Pattern: Ensuring a Single Source of Truth
Use Case: A streaming platform’s recommendation engine relies on a single configuration that is shared across multiple modules, such as movie suggestions and user preferences.
By using closures, functional programming allows the creation of a singleton instance that ensures the configuration is initialized only once. This prevents duplication and ensures consistency throughout the application.
3. Strategy Pattern: Dynamic Selection of Algorithms
Use Case: A payment gateway system needs to support multiple payment methods like credit cards, PayPal, and cryptocurrency. Depending on the user’s choice, a specific payment processing strategy must be applied.
In functional programming, strategies can be implemented as separate functions and dynamically passed as arguments to a higher-order function. This approach reduces coupling and makes it easier to add new strategies without modifying existing code.
4. Observer Pattern: Real-Time Updates
Use Case: A stock trading platform needs to notify users in real-time whenever stock prices change. Multiple modules, such as charts, notifications, and portfolio summaries, need to react to these changes.
Functional programming’s reliance on callbacks and event streams aligns perfectly with the observer pattern. Observables can be created as functions that register listeners and notify them when data changes, enabling a clean and scalable implementation.
5. Decorator Pattern: Extending Functionality
Use Case: An e-commerce platform allows users to apply multiple types of discounts (e.g., seasonal discounts, coupon codes, and loyalty points) to their purchases. These discounts should be composable and extendable.
Functional programming’s ability to compose functions allows decorators to wrap existing functionality dynamically. For example, different discount functions can be chained together to calculate the final price, making the solution both flexible and reusable.
6. Chain of Responsibility Pattern: Flexible Request Handling
Use Case: In a customer support system, user queries need to pass through multiple layers (e.g., chatbot, FAQ search, and human agent). Each layer should decide whether to handle the query or pass it to the next.
Functional programming enables chaining functions in a pipeline. Each function decides whether to process the input or pass it to the next function, providing a clean implementation of the chain of responsibility pattern.
7. Command Pattern: Simplifying Undo and Redo Operations
Use Case: A graphic design tool allows users to perform actions like drawing, resizing, and deleting shapes. Users should also be able to undo and redo these actions.
In functional programming, commands can be represented as data objects that store the necessary information to execute, undo, or redo actions. These objects are passed to functions responsible for handling them, ensuring that the system remains decoupled and flexible.
Key Takeaways
Design patterns are not restricted to object-oriented programming. With functional programming, you can reimagine these patterns to fit modern, declarative, and composable paradigms. TypeScript provides the perfect bridge for combining the best of both worlds, offering the type safety and structure of object-oriented approaches with the elegance and simplicity of functional programming.
By understanding and applying functional design patterns, you can build robust applications that are not only easy to maintain but also scalable and efficient. Whether it’s handling dynamic algorithms, ensuring real-time updates, or managing shared state, functional programming in TypeScript equips you to tackle complex challenges with confidence.
Leave a Comment
No comments yet. Be the first to comment!